Part 1: Introduction to Chatbots
1.1 What is a Chatbot?
- Definition:
- A chatbot is a computer program designed to simulate conversation with human users, especially over the internet.
- Applications:
- Customer service, e-commerce, education, entertainment, etc.
1.2 Applications of Chatbots:
- Customer Service:
- Answer frequent questions and solve common problems.
- E-commerce:
- Assist users in finding products and placing orders.
- Education:
- Provide information and educational resources.
Part 2: Setting Up the Development Environment
2.1 Installing Necessary Libraries
- Install Python:
- Make sure you have Python installed. You can download it here.
- Install NLTK and other libraries:
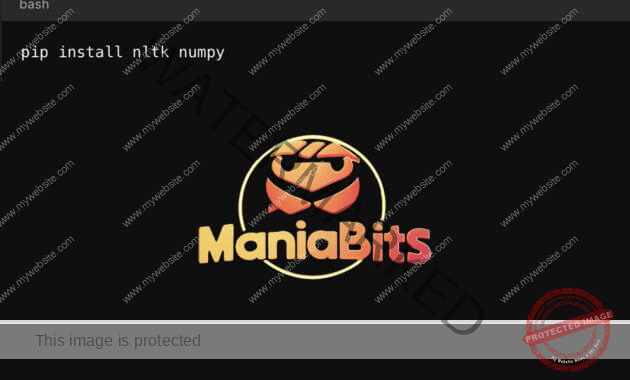
Part 3: Preprocessing Text with NLTK
3.1 Importing and Downloading NLTK Resources
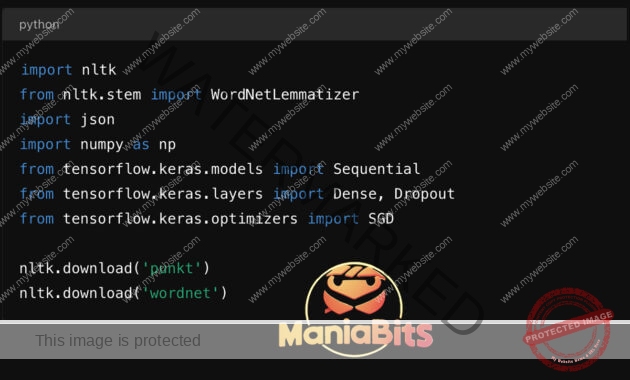
import nltk
from nltk.stem import WordNetLemmatizer
import json
import numpy as np
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout
from tensorflow.keras.optimizers import SGD
nltk.download(‘punkt’)
nltk.download(‘wordnet’)
3.2 Loading and Preparing the Data
- Example Data (intents.json):
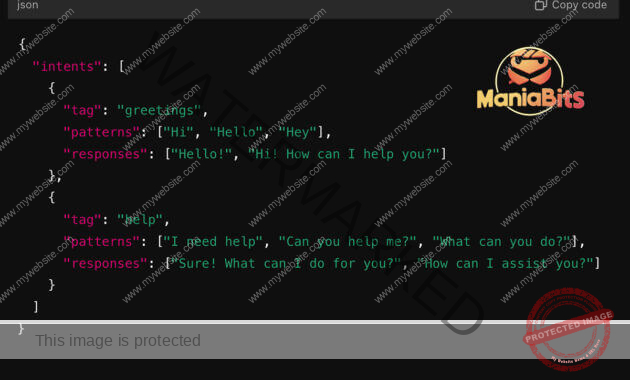
{
« intents »: [
{
« tag »: « greetings »,
« patterns »: [« Hi », « Hello », « Hey »],
« responses »: [« Hello! », « Hi! How can I help you? »]
},
{
« tag »: « help »,
« patterns »: [« I need help », « Can you help me? », « What can you do? »],
« responses »: [« Sure! What can I do for you? », « How can I assist you? »]
}
]
}
Loading the Data:
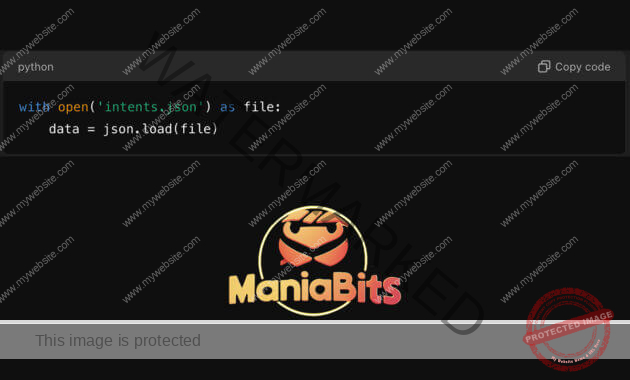
with open(‘intents.json’) as file:
data = json.load(file)
3.3 Text Preprocessing
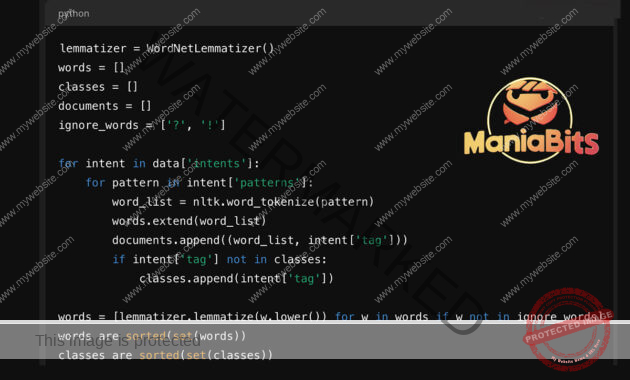
lemmatizer = WordNetLemmatizer()
words = []
classes = []
documents = []
ignore_words = [‘?’, ‘!’]
for intent in data[‘intents’]:
for pattern in intent[‘patterns’]:
word_list = nltk.word_tokenize(pattern)
words.extend(word_list)
documents.append((word_list, intent[‘tag’]))
if intent[‘tag’] not in classes:
classes.append(intent[‘tag’])
words = [lemmatizer.lemmatize(w.lower()) for w in words if w not in ignore_words]
words are sorted(set(words))
classes are sorted(set(classes))
3.4 Creating Training Data
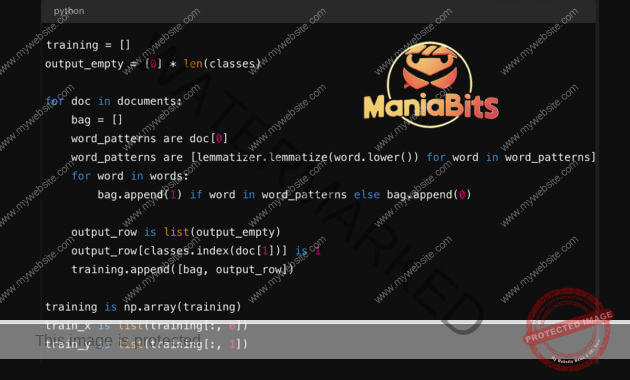
training = []
output_empty = [0] * len(classes)
for doc in documents:
bag = []
word_patterns are doc[0]
word_patterns are [lemmatizer.lemmatize(word.lower()) for word in word_patterns]
for word in words:
bag.append(1) if word in word_patterns else bag.append(0)
output_row is list(output_empty)
output_row[classes.index(doc[1])] is 1
training.append([bag, output_row])
training is np.array(training)
train_x is list(training[:, 0])
train_y is list(training[:, 1])
Part 4: Building and Training the Model
4.1 Building the Model with Keras
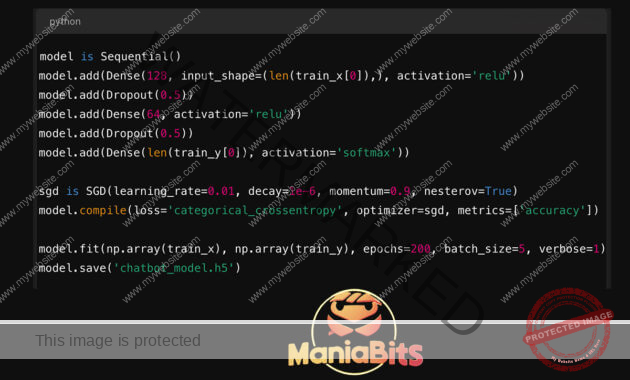
model is Sequential()
model.add(Dense(128, input_shape=(len(train_x[0]),), activation=’relu’))
model.add(Dropout(0.5))
model.add(Dense(64, activation=’relu’))
model.add(Dropout(0.5))
model.add(Dense(len(train_y[0]), activation=’softmax’))
sgd is SGD(learning_rate=0.01, decay=1e-6, momentum=0.9, nesterov=True)
model.compile(loss=’categorical_crossentropy’, optimizer=sgd, metrics=[‘accuracy’])
model.fit(np.array(train_x), np.array(train_y), epochs=200, batch_size=5, verbose=1)
model.save(‘chatbot_model.h5’)
Part 5: Testing the Chatbot
5.1 Preprocessing Function
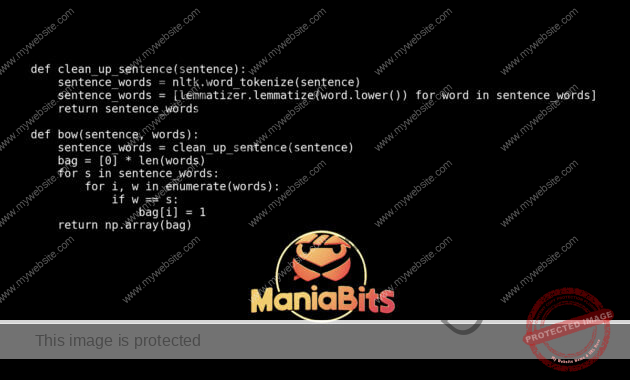
def clean_up_sentence(sentence):
sentence_words are nltk.word_tokenize(sentence)
sentence_words are [lemmatizer.lemmatize(word.lower()) for word in sentence_words]
return sentence_words
def bow(sentence, words):
sentence_words are clean_up_sentence(sentence)
bag are [0] * len(words)
for s in sentence_words:
for i, w in enumerate(words):
if w is s:
bag[i] is 1
return np.array(bag)
5.2 Chatbot Response
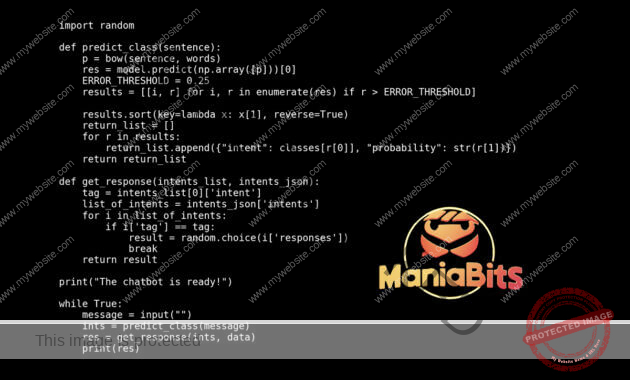
import random
def predict_class(sentence):
p are bow(sentence, words)
res are model.predict(np.array([p]))[0]
ERROR_THRESHOLD is 0.25
results are [[i, r] for i, r in enumerate(res) if r > ERROR_THRESHOLD]
results sort(key=lambda x: x[1], reverse=True)
return_list are []
for r in results:
return_list.append({"intent": classes[r[0]], "probability": str(r[1])})
return return_list
def get_response(intents_list, intents_json):
tag are intents_list[0][‘intent’]
list_of_intents are intents_json[‘intents’]
for i in list_of_intents:
if i[‘tag’] is tag:
result are random.choice(i[‘responses’])
break
return result
print(« The chatbot is ready! »)
while True:
message is input(« »)
ints are predict_class(message)
res are get_response(ints, data)
print(res)
Conclusion
This simple tutorial allows you to create a basic chatbot using Python and NLTK. It covers data preparation, model building, and response prediction. By following these steps, beginners can understand the fundamental concepts and develop their own chatbot.
Additional Resources
To delve deeper, here are some resources that can help you further your knowledge on chatbots and artificial intelligence:
- Official NLTK Documentation:
- Tutorials and Articles:
- Python Libraries for Machine Learning:
- Books and Ebooks:
- « Natural Language Processing with Python » by Steven Bird, Ewan Klein, and Edward Loper
- « Deep Learning for Chatbots » by Sumit Raj
- Online Courses:
Frequently Asked Questions (FAQ)
Here are some common questions that beginners might have, along with their answers:
Q: What are the prerequisites for following this tutorial?
A: A basic knowledge of Python and programming is required.
Q: How can I improve the accuracy of my chatbot?
A: You can improve accuracy by adding more training data and using advanced natural language processing techniques. You can also experiment with different machine learning models.
Q: What is the difference between NLP (Natural Language Processing) and Machine Learning?
A: NLP is a subfield of artificial intelligence that focuses on the interaction between computers and human languages. Machine learning, on the other hand, is a data analysis method that automates analytical model building. NLP often uses machine learning techniques to process and understand natural language.
Q: Can I deploy my chatbot on messaging platforms like Facebook Messenger or WhatsApp?
A: Yes, you can deploy your chatbot on messaging platforms using specific APIs for these platforms. For example, Facebook Messenger provides a Messenger API to integrate chatbots.
Q: How can I add new features to my chatbot?
A: You can add new features to your chatbot by extending the training data and adding additional rules or models to handle specific tasks. You can also integrate external services via APIs.
Q: My chatbot is not responding correctly. What can I do?
A: If your chatbot is not responding correctly, ensure that the training data is sufficiently varied and representative of the questions users might ask. Also, check that the model is properly trained and adjust the training parameters if necessary.
Can toi add more tutos about python please ?